Machine Learning (ML) in Bioinformatics
Algorithm Complexity
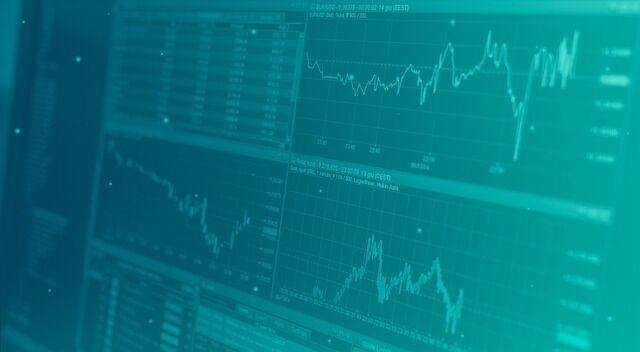
Learning objectives:
Introduction to Algorithm Complexity
Algorithm complexity measures an algorithm's efficiency in terms of time and space, compares different algorithms, and determines the most efficient for any given problem. We can divide algorithm complexity into two main categories: time complexity and space complexity.
Algorithm complexity is an important concept to consider when designing algorithms. It helps determine the efficiency of an algorithm in terms of time and space and can be used to compare different algorithms. The two main algorithm categories are time and space complexity and can be expressed using Big O notation. Additionally, average-case and worst-case complexity should also be considered when designing algorithms.
Time Complexity
Time complexity is the amount of time it takes for an algorithm to execute its tasks. It is typically measured in terms of the number of operations or steps that must be taken to complete the algorithm.
The time complexity of an algorithm is determined by analyzing the number of operations it performs and the time required for each operation. Time complexity is usually expressed in Big O notation, which expresses the relationship between the input size and the running time of an algorithm.
Space Complexity
Space complexity is the amount of memory or storage an algorithm requires to execute its tasks. It is typically measured in terms of the number of additional data structures or variables an algorithm needs to store to function.
Space complexity is vital when designing algorithms, as it can impact their overall performance. Space complexity is also typically expressed in Big O notation, which expresses the relationship between the input size and the amount of memory or storage an algorithm needs.
Average and Worst-Case Complexity
When discussing algorithm complexity, it is essential to consider average-case and worst-case complexity. Average-case complexity is the expected running time of an algorithm, given a particular set of inputs. It is typically used to compare different algorithms in their expected performance.
Worst-case complexity is the maximum running time of an algorithm, given a specific set of inputs. It is essential to consider when designing algorithms, as it can help determine the feasibility of an algorithm for a specific problem.
Analysis of Algorithm Complexity
Types of Complexity Analysis
Several types of time complexity analyses can be used to evaluate an algorithm's efficiency.
The most common type is average-case analysis, which looks at the average amount of time it takes an algorithm to complete a task. The average-case analysis is often used when analyzing algorithms that have random inputs.
The worst-case analysis looks at the maximum amount of time it takes an algorithm to complete a task. This analysis is often used when analyzing algorithms that have deterministic inputs.
The best-case analysis looks at the minimum amount of time it takes an algorithm to complete a task. This analysis is often used when analyzing algorithms that have deterministic inputs.
The amortized analysis looks at the total cost of an algorithm over multiple runs. This analysis is often used when analyzing algorithms that perform multiple tasks.
Analyzing Time Complexity
When analyzing an algorithm's time complexity, you must first identify the type of analysis you will use. The type will depend on the type of inputs the algorithm has and the type of analysis you want to do.
Once you have identified the type of analysis you will use, you need to determine the algorithm's time complexity. The time complexity can be determined by calculating the number of operations the algorithm performs and then expressing the time complexity using Big O notation.
For example, consider an algorithm that takes an array of \(n\) elements and sorts them in ascending order. To calculate the time complexity of this algorithm, we need to count the number of operations it performs. In this case, the algorithm performs \(n\) operations for each element in the array, so the time complexity is \(O(n)\).
Once you have calculated the time complexity of an algorithm, you can compare it to other algorithms to determine which one is more efficient. For example, if you have two algorithms with the same input size but one has a time complexity of \(O(n^2)\) and the other has a time complexity of \(O(n)\), then the latter is more efficient.
Analyzing Space Complexity
Analyzing space complexity is a fundamental skill for any computer scientist, and it helps determine the amount of memory an algorithm will require to run efficiently. Analyzing space complexity can be tricky, especially with more advanced algorithms, but the basics are relatively straightforward.
What is Space Complexity?
Space complexity measures the amount of memory an algorithm uses in its execution. In other words, it is the amount of memory an algorithm requires to solve a problem. Memory can refer to both main memory (RAM) and secondary storage (disk). Space complexity is usually expressed as a function of the input's size, denoted by \(n\).
The Basics
When analyzing space complexity, you typically start by looking at the code and identifying the variables used in the algorithm. The size of the variables will determine the amount of memory required for the algorithm. For example, if you have an array of length \(n\), then the space complexity will be \(O(n)\). If you have a matrix of size \(m \) x \(n\), then the space complexity will be \(O(mn)\).
An excellent way to think about space complexity is to consider the number of operations that must be performed to solve the problem. For example, if you have a loop that runs \(n\) times, then the space complexity will be \(O(n)\). However, if you have a loop that runs 2\(n\) times, the space complexity will be \(O(2n)\).
Advanced Techniques
Once familiar with analyzing space complexity, you can move on to more advanced techniques. One common technique is to consider the data structures used in the algorithm.
Different data structures have different space complexities. For example, a linked list has a space complexity of \(O(n)\), while a binary tree has a space complexity of \(O(\log n)\).
Another essential technique is to consider the total amount of memory allocated by the algorithm. The total amount of memory includes the variables declared in the code and any data structures created during the execution of the algorithm. This analysis can help you identify potential memory leaks in your code.
Applying Big O Notation
Big O notation is one of computer science's most commonly used tools, and it is used to analyze algorithms and compare the performance of different algorithms.
What is Big O Notation?
Big O notation is a notation used to represent the asymptotic behavior of a function. It tells us how the running time of a function grows as the size of the input grows. It can be used to analyze the running time of algorithms by measuring the number of operations (or steps) they need to complete their task.
For example, consider the following algorithm:
1. Start with an array of integers.
2. Loop through the array and add each integer to a sum.
3. Return the sum.
The above algorithm has a time complexity of \(O(n)\), where \(n\) is the element count in the array. The notation means that the running time of this algorithm is proportional to the input size.
How to Apply Big O Notation?
When applying Big O notation, you need to understand the concept of “time complexity.” Time complexity measures how long it takes for an algorithm to complete its task, and this concept is measured in terms of the number of operations (or steps) the algorithm needs to complete its task.
There are the main types of time complexity:
- \(O(1)\):
- Constant time complexity. This means that the algorithm takes the same time regardless of the input size.
- \(O(n)\):
- Linear time complexity. This means that the algorithm takes a linear amount of time with respect to the size of the input.
- \(O(\log n)\):
- Logarithmic time complexity. This means that the algorithm takes a logarithmic amount of time with respect to the size of the input.
- \(O(2^n)\):
- Exponential time complexity. This means that the algorithm takes an exponential amount of time with respect to the size of the input.
Once you understand the concept of time complexity, you can begin to apply Big O notation. The basic syntax of Big O notation is \(O(f(n))\), where \(f(n)\) is a function of n (the size of the input). For example, if an algorithm takes a linear amount of time to complete its task, its time complexity is \(O(n)\).
To determine the time complexity of an algorithm, you need to look at the operations (or steps) it performs. If the algorithm does a specific operation once for every element in the input, it has a linear time complexity \((O(n))\). If the algorithm does a particular operation twice for every element in the input, it has a quadratic time complexity \((O(n^2))\).
Summary
Big O notation is a notation that we use in computer science. It describes the performance or complexity of an algorithm. It is used to analyze the running time of algorithms by measuring the number of operations (or steps) they need to complete their task.
o apply Big O notation, you need to understand the concept of time complexity and how it relates to the algorithm you are analyzing. Once you understand the concept of time complexity, you can use Big O notation to determine the time complexity of an algorithm.
Techniques for Improving Algorithm Complexity
In computer programming and software engineering, performance bottlenecks can significantly impact the speed and efficiency of an algorithm. Performance bottlenecks are areas of an algorithm with a significant slowdown in the execution of code or a major increase in resource usage. These bottlenecks can drastically limit the performance of an algorithm, making it both slow and inefficient. Identifying and resolving performance bottlenecks is one of the most critical tasks in optimizing algorithms.
The first step in identifying performance bottlenecks is to analyze the algorithm. This analysis involves examining the algorithm's structure and performance characteristics to identify potential issues.
It is helpful to think about the algorithm's overall structure when analyzing algorithms. This includes determining the number of iterations, loops, and other elements that make up the algorithm. It is also important to consider the data structures used to store and manipulate the data used by the algorithm.
In addition to structural analysis, it is also essential to consider the algorithmic complexity of the algorithm.
The structural analysis includes analyzing the time complexity (i.e., how long it takes to execute) and the space complexity (i.e., how much memory it takes to execute). Understanding the complexity of an algorithm is key to identifying potential bottlenecks.
Finally, it is vital to consider the performance characteristics of the algorithm. The performance characteristics include looking at the runtime behavior of the algorithm, including execution times and memory usage. Analyzing the performance metrics can help to identify areas of the algorithm which may be causing performance issues.
Common Performance Bottlenecks
Once an algorithm has been analyzed, it is possible to identify common performance bottlenecks. Some of the most common performance bottlenecks include:
Unnecessary Computation: This occurs when an algorithm performs unnecessary computations, resulting in increased execution time and memory usage.
Excessive Memory Usage: This occurs when an algorithm requires more memory than necessary, resulting in decreased performance.
Unnecessary Data Access: This occurs when an algorithm accesses unnecessary data, resulting in increased execution time and memory usage.
Poor Data Structures: This occurs when an algorithm uses an inefficient data structure, resulting in increased execution time and memory usage.
Strategies for Resolving Performance Bottlenecks
Once potential bottlenecks have been identified, developing strategies for resolving them is essential. Common strategies for resolving performance bottlenecks include:
- Optimizing Data Structures:
- Optimizing data structures can reduce the time and memory required to execute an algorithm. Standard techniques include selecting the most efficient data structure for a given task, reducing the size of data structures, and caching data.
- Optimizing Algorithms:
- Optimizing algorithms can reduce the time and memory required to execute an algorithm. Standard techniques include reducing the number of iterations, reducing the size of data structures, and reordering instructions.
- Parallelizing Algorithms:
- Parallelizing algorithms can reduce the time required to execute an algorithm by running multiple threads simultaneously. Standard techniques include using thread pools, dividing tasks into subtasks, and using distributed computing.
Identifying and resolving performance bottlenecks is an essential part of optimizing algorithms. Analyzing the algorithm's structure and performance characteristics makes it possible to identify potential bottlenecks.
Once identified, common strategies such as optimizing data structures, optimizing algorithms, and parallelizing algorithms can be used to resolve them. With the right approach, it is possible to improve the performance of an algorithm significantly.
Utilizing Data Structures
Data structures are one of the most critical components of efficient algorithms. Without properly utilizing data structures, algorithms can quickly become bloated and complicated.
Understanding the different types of data structures and how to use them effectively is key to improving algorithm complexity and achieving efficient results. In this tutorial, we will discuss various techniques for utilizing data structures in order to improve algorithm complexity.
What are Data Structures?
Data structures are collections of data elements (values or variables) organized for efficient access and manipulation. They are used to store, organize, and efficiently retrieve data.
Data structures are often divided into two categories: linear and non-linear. Linear data structures, such as arrays and linked lists, are composed of elements that are organized linearly according to some order. Non-linear data structures, for example, as trees and graphs, contain elements that are not organized linearly and instead form a structure that can be navigated and traversed.
Why Utilize Data Structures?
Utilizing data structures is essential for improving the complexity of algorithms. Data structures allow algorithms to access and manipulate data efficiently.
For example, suppose an algorithm needs to look up a specific value from extensive data collection. In that case, it can quickly utilize a data structure, for example, a hash table, to locate the desired value. Utilizing data structures also helps to reduce the amount of code needed to implement an algorithm, thus reducing the overall complexity.
Techniques for Utilizing Data Structures
There are several techniques for utilizing data structures to improve algorithm complexity. These techniques include:
Divide and Conquer
One of the most popular techniques for utilizing data structures is divided and conquer. Divide-and-conquer algorithms divide a problem into smaller, manageable subproblems and then solve each subproblem individually. This approach can reduce the overall complexity of an algorithm by breaking down a large problem into smaller ones.
For example, the quicksort algorithm is a divide-and-conquer algorithm that sorts an array of elements by recursively dividing the array in half and sorting each half.
The quicksort algorithm can achieve better overall complexity than other sorting algorithms by breaking down the problem into smaller pieces.
Greedy Algorithms
Greedy algorithms are algorithms that make decisions based on the current state of the data. They utilize data structures such as priority queues and heaps to ensure that the most important values are always available at the top of the structure. This allows the algorithm to quickly decide which action to take based on the current data set.
Graph Traversal
Graphs are a type of non-linear data structure that can be used to represent relationships between objects. Graphs can be utilized to solve complex problems by traversing the graph and visiting each node in the graph. By utilizing data structures such as adjacency lists, algorithms can quickly traverse graphs and find solutions to complex problems.
Hashing
Hashing is a technique used to map data elements to specific locations in a data structure. This allows the algorithm to quickly locate a specific value in a large dataset without having to search through the entire data structure. Hashing utilizes data structures such as hash tables and maps to store, organize, and retrieve data efficiently.
Dynamic Programming
Dynamic programming is a technique for improving the efficiency of an algorithm by avoiding recomputing solutions to previously solved subproblems.
Instead of recalculating a solution to a subproblem, dynamic programming stores the solutions to previously solved subproblems and uses them to solve the current problem. This technique can reduce the complexity of an algorithm by avoiding unnecessary calculations and reducing the amount of time and memory needed to complete a task.
By understanding the different data structures and how to use them effectively, algorithms can quickly and efficiently access and manipulate data.
Parallelizing Algorithms
Parallelizing algorithms is a technique used to improve algorithm complexity by executing multiple instructions simultaneously. It can be used to increase the speed and efficiency of an algorithm, as well as reduce its memory consumption.
This technique is beneficial for algorithms that require a lot of processing power and would otherwise take a long time to run. We will discuss the various techniques for parallelizing algorithms and how we can use them to improve algorithm complexity.
What is Parallelization?
Parallelization is splitting an algorithm into multiple parts and executing them concurrently. Parallelization of algorithms allows for more efficient use of computing resources and can reduce the time required to complete a computation. The same task can be completed using multiple processors or threads in a fraction of the time it would take with a single processor or thread.
How Does Parallelization Work?
Parallelization works by dividing a task into smaller tasks and assigning each task to a different processor or thread. Each processor or thread then executes its assigned task independently of the others. Once we have completed all tasks, we combine the results to produce the final output.
Types of Parallelization
Several types of parallelization techniques can be used to improve algorithm complexity. These include:
Data Parallelism
Data parallelism is a technique where multiple copies of the same data set are processed in parallel. This allows for faster processing by taking advantage of multiple processors or threads.
Task Parallelism
Task parallelism is a technique where multiple tasks are split into multiple threads and executed in parallel. This allows faster processing by distributing the load across multiple processors or threads.
Instruction-Level Parallelism
Instruction-level parallelism is a technique where multiple instructions within a program are executed in parallel. This allows for faster execution by taking advantage of multiple processors or threads.
Benefits of Parallelization
The main benefit of parallelization is that it can enormously reduce the time required to complete a task. By utilizing multiple processors or threads, the same task can be completed in a fraction of the time it would take with a single processor or thread.
Additionally, parallelization can reduce the amount of memory required to store data by breaking down larger datasets into smaller chunks that can be stored in multiple locations.
Summary
Algorithm complexity is a measure of the efficiency and effectiveness of an algorithm, and it is a way to compare algorithms and make predictions about their performance. Algorithm complexity can be measured in terms of time, space, and other resources.
Time complexity measures the time it takes for an algorithm to complete its task. Many algorithms take a long time to complete depending on the input data size. For example, if the input data is large, the algorithm might take more time to complete. Time complexity is usually expressed using Big-O notation, which gives an upper bound on the running time of an algorithm.
Space complexity measures the amount of space an algorithm needs to store data. Space complexity is important because it affects how efficiently an algorithm can use memory. Algorithms with low space complexity are more efficient than algorithms with high space complexity because they require less memory to store their data. Space complexity is usually expressed using Big-O notation, which gives an upper bound on the space used by an algorithm.
The complexity of an algorithm can also depend on other factors, such as the number of operations performed, the number of comparisons made, or the number of variables used. The complexity of an algorithm is also affected by the structure of the data. For example, if the data is structured to make it easy to access certain elements, then the algorithm will be more efficient.
Finally, the complexity of an algorithm can be affected by the programming language used to implement it. Different programming languages have different levels of complexity so the same algorithm might run faster or slower depending on the language used.
Overall, algorithm complexity is vital to understand when designing and analyzing algorithms. It helps to identify the best algorithms for a given problem and can also be used to make predictions about the performance of an algorithm. Knowing an algorithm's complexity can help optimize its implementation and improve performance.